Web Application Development
In this ”how to create a web app” tutorial, we’ll learn how to create a web app using a combination of several development technologies including ASP .NET MVC, C# Razor and Visual Studio. Let’s get to it!
Defining Concepts
What is ASP.NET?
Microsoft ASP.NET is a framework used in web application development, dynamic websites and web services. There have been 6 frameworks so far since this technology was created: 1.0, 1.1, 2.0, 3.5, 4.0 and the current version 4.5. Every framework has been adding more and more classes, methods, types, etc., so programmers are able to do more things using these new features.
What is MVC?
MVC is a software architecture pattern which separates the development of applications into three concepts or components: Model, View and Controller. As you can see, this is where the name comes from. Each component manages and represents a particular aspect of the application:
- Model - It manages the entity definition, data access and business logic of our application.
- View - It represents the user interface and where the user can see the information provided by the model.
- Controller - It’s how the Model and View components communicate or interact. It’s the link between the two.
MVC is a pattern so it's not exclusive of any company or license. There are many MVC frameworks. For example, Cocoa, using Objective C licensed by Apple, Ruby On Rails, using Ruby licensed by MIT and Grails using JAVA licensed by APACHE. We could continue with so much more, but the one we are focusing on in this tutorial is Microsoft ASP.NET MVC, using C#. Currently, there are 4 versions of ASP.NET MVC and in this tutorial, we’ll use MVC 3.
What is C#?
C# is an object-oriented programming language developed by Microsoft as part of the .NET framework. . A C# web application will run on the Windows ecosystem and there is a lot that can be leveraged for a powerful, seamless experience.
What is Razor?
Razor is an ASP.NET programming syntax that allows the programmers to write some C# or Visual Basic code embedded in an html page and is used to create dynamic web pages. It was released by Microsoft for Visual Studio 2010 in January 2011 as part of ASP.NET MVC 3.
What is Visual Studio?
It is a tool, or technically speaking, an Integrated Development Environment (IDE) used to create applications of several kinds such as windows form applications, web sites, web applications and web services. It supports different programming languages like C#, as well as Visual Basic, C, C++, and provides support for Python and Ruby.
Let’s Start Developing
Now that we know a little more about these software development technologies and we have all our ingredients, let’s mix them.
1. Open Visual Studio 2012, go to "File/New" menu and click on "Project". A window like the following will open:
2. In this window, select within the Visual C# options, ASP.NET MVC 3 Web Application.
3. Then in the following window, select the template “Empty”, and “Razor” as View engine, and then click Ok.
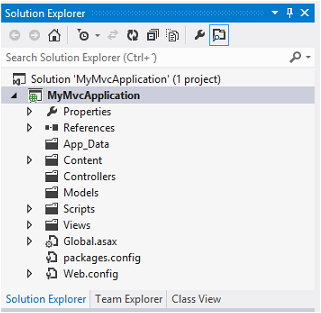
4. Now right-click on the folder “Controllers” and "Add/Controller” and you’ll see a window where you can enter the name of your controller. Let’s name it “Index”, and for the template option let’s leave Empty Controller. Don’t remove the suffix “Controller” in the controller name because it’s indicating that this one is a controller class.
5. Now, right-click on the folder “Models” and select Add/Class. Name this class whatever you want as this will be the Entity to show in the View. Let’s name it “IndexModel” (the name doesn’t have to include the word “index”). Then, add some properties to the class. For this example, let’s add ID, Description and Comments properties.
using System; namespace MyMvcApplication.Models } |
6. Now, right-click on the folder “Views” and create a new folder named “Index” (as the controller). Then right-click on that folder and select Add/View. Let’s name the view as “Index” also and as a result, we’ll have a new “Index. cshtml”.
7. Let’s add some code to the controller which will look like this:
8. Now, let’s add some code to the view which will look like this.
9. Now, run the Project using your favorite browser, and make sure you add to the URL the action “Index”.
10. That’s it! We have our first MVC Application with ASP.NET MVC 3, using C# and Razor.
Final Thoughts
I hope you enjoyed this ASP .NET MVC tutorial. MVC is an interesting software development technology that allows you to organize our applications using these three layers. Therefore, it is a good approach when every member of a development team works only with part of the code, like the business logic for example (Back-End), and other developers work only with Front-End.
However, just as there are different programming languages to leverage for an excellent web application, there are also universal programming principles that help streamline projects. At iTexico, our teams strive for excellence and providing the best possible services. Learn more about our services today!
This article was updated for comprehension purposes on August 23rd 2018.
References:
About the Author
Rommel S. is a .NET developer with 7+ years of experience mostly in web applications. He likes analyzing, designing and developing applications and complex Information Systems.
Post Your Comment Here