Web App Development: Introduction to GRUNT, a JavaScript Task Runner
Hey front-end developers! When you are building a web app and working on a JavaScript project, there are a bunch of things you’ll want to do regularly. For example you would like to perform tasks like, minification, compilation, unit testing, linting, etc. After you have configured it, a task runner can do most of that work for you. All those tasks are configured in a JavaScript file called Gruntfile.js that makes it really easy to get started and you will be happy watching how easy your job becomes.
So why use a task runner? I could say it in just one word: Automation.
Why choose Grunt as the project's tasks runner?
Well, there are 4 main points that are very useful. Lets see:
Utility
Grunt.js allows you to run your tasks when it looks for its configuration under a property of the same name. The same way multi-tasks can have multiple configurations. It also allows you to create tasks using aliases and this is a very useful feature that lets you to abstract the detail of a generic task.
One example could be when you minify, test, concatenate, and abstract under a task named "build".
Consistency
Grunt.js provides you a consistent interface for configuring and using any task which means that it pushes you to work and use a unified set of commands, ensuring everyone that wants to configure grunt.js writes the code of the same standard.
Community
Grunt.js has a strong and a growing community of Front-End Web Developers. There is already a whole bunch of helpful tasks available for use today.
Power & Flexibility
Grunt.js provides all the potential of Node.js for your tasks, and as mentioned before it help you by automating the tasks.
And those are the 4 points to take into account. So now that we know a little about the basics, now you might be wondering how to intall Grunt. I'll show you how.
Installing Grunt
As you know Grunt is built on Node.js, and it's available as a package via the Node package manager (npm). So its very easy to install.
npm install grunt --save-dev |
Then install any Grunt Module you may need in your build process, for example:
npm install grunt-contrib-concat --save-dev |
Now let’s create a package.json and a Gruntfile.js in the root of our project.
package.json
So what exactly does package.json do? This file tells NPM which dependencies we will install for our project. This has lots of advantages and it helps you when you are working with a team by keeping all the environments in sync and use the same dependencies.
Run npm install, and NPM will go fetch these for us and place them in a node_modules folder.
One example of a package.json is:
{ "name": "my-project-name", "version": "1.0.0", "devDependencies": { "grunt": "~0.4.1", "grunt-contrib-compass": "~0.2.0", "grunt-contrib-watch": "~0.4.3", "grunt-contrib-uglify": "~0.2.0" } } |
Now you have to setup your Gruntfile.js that will describe your build process.
module.exports = function(grunt) {
// Project configuration. grunt.initConfig({ pkg: grunt.file.readJSON('package.json'), uglify: { options: { force: true } } });
// Load the plugin that provides the "uglify" task. grunt.loadNpmTasks('grunt-contrib-uglify');
// Default task(s). grunt.registerTask('default', ['uglify']);
}; |
Every Gruntfile uses a basic format named wrapper function, and all of your Grunt code must be specified inside this function:
module.exports = function(grunt) { // grunt code }; |
grunt.initConfig({});
grunt.initConfig initializes a task configuration object for projects that we are working on. You may store any arbitrary data inside of grunt.initConfig, as long as it doesn’t conflict with properties your tasks require otherwise it will be ignored.
Custom tasks
You can configure Grunt to run one or more tasks by default by defining a default task. Running grunt at the command line without specifying a task will run the uglify task. This is functionally is the same as explicitly running grunt uglify or even grunt default. Any number of tasks (with or without arguments) may be specified in the array, for example:
grunt.registerTask('default', ['uglify']); |
Companies and projects that are working with Grunt
These are several companies and projects that are working Grunt, for example Adobe, jQuery, Twitter, Bootstrap, Adobe, WordPress, Walmart and many others. Feel free to check it out and see if this tool fits your workflow and can make your work easier.
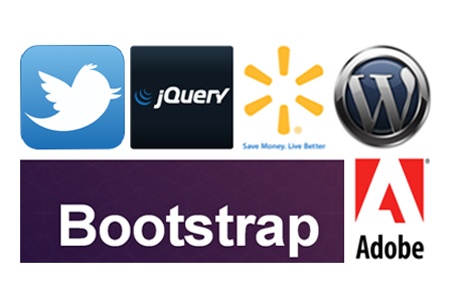
That's it for now and when you start to use Grunt for your projects, you will see what a great tool it is. For more inoformation about the task runner you can visit the official web page. Just click here.
About the Author
Javier Salazar is a Front End developer and considers himself a huge fan of the new javascript tools, improvements to actual js libraries and best practices. He currently works at iTexico as Front End developer.
Post Your Comment Here